Creating game objects at runtime in Unity3D
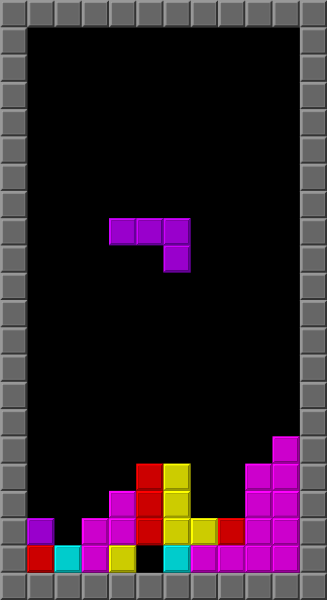
In this article, I will continue with another beginner topic. In the previous article, we talked about finding game objects at runtime. This time, I will write about how we create new game objects at runtime in Unity3D. In games, we always instantiate new game objects. These could be enemies, coins, obstacles, bullets, etc. Therefore, it is important to understand how these objects are created or in other words instantiated.
In Unity3D, in order to create new game objects, we use the method Instantiate( ). This method has several variations that take different numbers of parameters. In each of these variations, a clone of the first parameter is created.
Contents
- How to instantiate a prefab
- Position and rotation issues
- How to instantiate a prefab from the resources folder
- How to create primitives like a cube in runtime
- How to create an empty game object
- Instantiating random objects
How to instantiate a prefab
In this section, we will see how we instantiate a game object that has not an instance in the scene. But before that let me explain what prefabs are, since we use them for instantiation.
A prefab is something like a blueprint. Prefabs allow us to prepare an object for the scene and thus, we can create instances of these prefabs during runtime or directly in the editor. They are useful especially when we want to modify these objects. Just modifying the prefab properties will affect each game object that is created from this prefab.
To create a prefab, prepare your game object in the Hierarchy and just drag and drop it to the Project tab as in the following video.
Now, we can use this prefab to add this object to our scene either in the editor or in runtime. To add this prefab in the editor, just drag the prefab and drop to the scene. You can add as many objects as you want. On the other hand, to create an instance of it in runtime, keep reading.
As mentioned, we use the method Instantiate( ) to create a new game object at runtime. For instance, the following script instantiates the robotPrefab when we press the key C on the keyboard.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { public GameObject robotPrefab; void Update() { if(Input.GetKeyDown(KeyCode.C)){ Instantiate(robotPrefab); } } }
You can add this script to any game object in the scene but I created an empty game object and add it. Your script in the inspector should look like this:
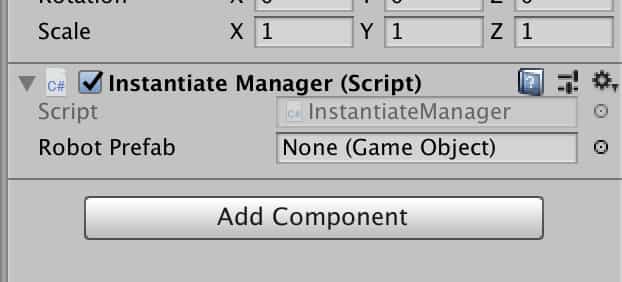
Now, we can assign the prefab that we would like to instantiate at runtime to the Robot Prefab slot.
Whenever we press key C on the keyboard, the assigned prefab will be created.
Since we did not specify an instantiation point, each of the robots created in the same place, and because of this, we cannot see them. We will see how we instantiate them at the location that we specify below but before that let’s talk about changing properties of the instantiated object.
For instance, let’s change the name of the newly created object. To do this, we only need to assign the Instantiate( ) method to a GameObject variable like the following:
GameObject instantiatedObject = Instantiate(robotPrefab);
Hence, we can access any property of the instantiated object. The next script creates a new object and changes its name:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { public GameObject robotPrefab; int i = 0; void Update() { if(Input.GetKeyDown(KeyCode.C)){ GameObject instantiatedObject = Instantiate(robotPrefab); instantiatedObject.name = "Robot " + i.ToString(); i++; } } }
Position and rotation issues
In the last section the object, that we created, could not be seen since all of them are created at the same place. We can determine the position and the rotation of the game object that we want to instantiate. To do this, we need to add second and third parameters to the instantiate method. While the second parameter determines the position, the third parameter determines the rotation of the object.
Instantiate(GameObject prefab, Vector3 position, Quaternion rotation);
Here, the position is in the world space, and the rotation represented by a quaternion. If you are reading this blog post, probably, this is the first time that you encounter the term quaternion. Let me explain briefly what a quaternion is. Quaternion is a representation of a rotation. In unity, quaternions are used intrinsically in physical and mathematical calculations. If you are not familiar with quaternions, please don’t be afraid. You don’t have to know the quaternions in detail. The only thing that you have to know is that quaternions are representations of rotations. It is very easy to convert a rotation which is given by Euler angles to quaternions.
The following script instantiates the same object by shifting 0.5 units every time in the x-direction and rotating 30 degrees about y-axis.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { public GameObject robotPrefab; int i = 0; void Update() { if(Input.GetKeyDown(KeyCode.C)){ GameObject instantiatedObject = Instantiate(robotPrefab, new Vector3(i*0.5f, 0f, 0f), Quaternion.Euler(0f, i*30f, 0f)); instantiatedObject.name = "Robot " + i.ToString(); i++; } } }
How to instantiate a prefab from the resources folder
Sometimes you may need to load a prefab directly from the resources folder. To do this, you should locate your prefab under the resources folder and just use the method Resources.Load( ).
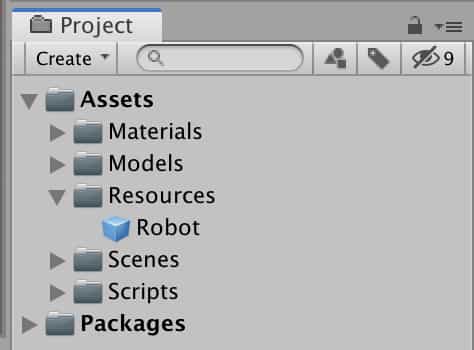
For instance, in the following script, when we press the key C on the keyboard, Robot prefab in the Resources folder loads, and thus, we can instantiate it.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { void Update() { if(Input.GetKeyDown(KeyCode.C)){ GameObject robotPrefab = (GameObject)Resources.Load("Robot"); Instantiate(robotPrefab); } } }
Observe that we have to cast the Resources.Load( ) method as GameObject since it could be in any type. In other words, we can get anything and load it into our scene in the Resources folder. It can be a Texture, it can be a material or anything like that. Since we want to load a game object we have to declare it as a GameObject. If it was a material, we would definitely need to cast as Material.
Another thing that you should keep in mind that the string parameter which is taken by the Resources.Load( ) method, indicates the relative path. For example, if your prefab is under another folder in the Resources folder, then you need to write the method as the following:
GameObject robotPrefab = (GameObject)Resources.Load("SomeFolder/Robot");
How to create primitives like a cube at runtime
In Unity3D, there are various primitive objects like cube, plane, sphere, or cylinder. You can add these objects either in the editor or using C# scripts at runtime.
To create these primitive game objects at runtime, we use the method GameObject.CreatePrimitive( ). This method creates the primitive object that is given as a parameter. The primitive geometric object will be created at the center of the scene. Therefore, you need to set its position or rotation if you would like to change its transform right after the object is created.
The following script will create a cube at the origin when you press the key C on the keyboard.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { void Update() { if(Input.GetKeyDown(KeyCode.C)){ GameObject cube = GameObject.CreatePrimitive(PrimitiveType.Cube); } } }
Other primitive types that you can create in Unity3D are given below.
GameObject.CreatePrimitive(PrimitiveType.Sphere); GameObject.CreatePrimitive(PrimitiveType.Cylinder); GameObject.CreatePrimitive(PrimitiveType.Plane); GameObject.CreatePrimitive(PrimitiveType.Capsule);
How to create an empty game object
In order to create an empty game object, the only thing that you need to do is to create an instance of GameObject using the new keyword.
GameObject obj = new GameObject( );
You can specify a name for the empty game object as well.
GameObject obj = new GameObject("Name of the game object")
Instantiating random objects
The best method of doing this is to create an array of prefabs. Then we can create the object by generating a random number and use this random number as the index of the prefab array.
For example, the following script will instantiate anyone of the objects that you specify in the array when you press the key C on the keyboard.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class InstantiateManager : MonoBehaviour { public GameObject[] prefabArray; int i = 0; void Update() { if(Input.GetKeyDown(KeyCode.C)){ int randomNumber = Random.Range(0, prefabArray.Length); Instantiate(prefabArray[randomNumber], new Vector3(i, 0f, 0f), Quaternion.identity); i++; } } }
In the script above, Quaternion.identity means there is no rotation on the object, and the variable i only to create objects next to the previous one.