How to find game objects in Unity3D
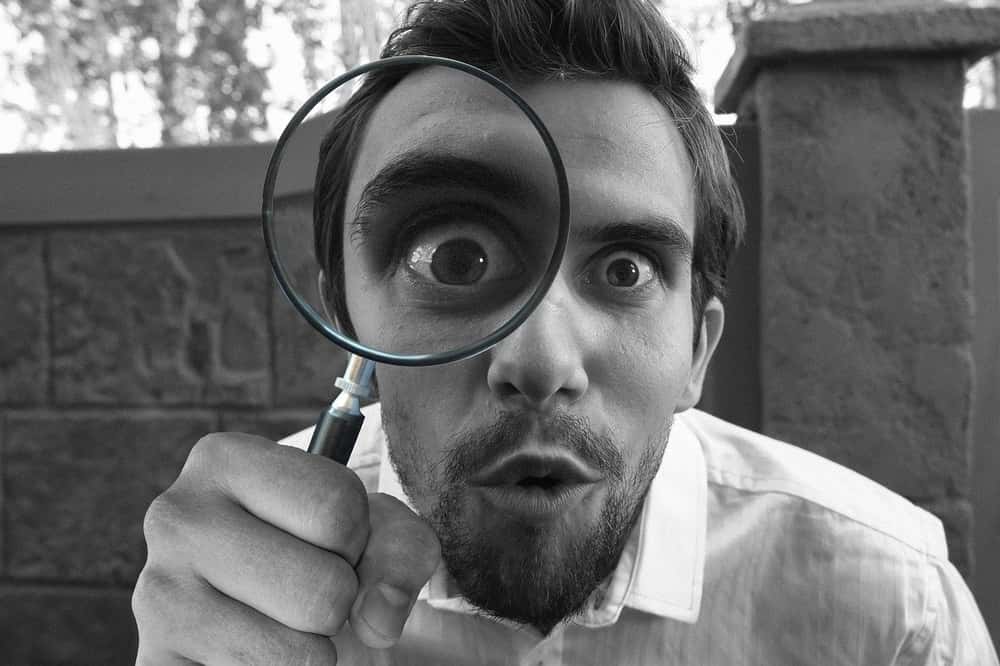
Finding and referencing a game object properly in Unity3D is one of the most asked questions for those who are new to Unity3D. In this article, I will write about referencing game objects, finding them according to their names, tags, and types. But let me give you the answer to the question at the title briefly.
In Unity3D, we can find game objects according to their names, tags, and types. For these purposes, we use the following methods respectively: GameObject.Find( ), GameObject.FindWithTag( ) and Object.FindObjectOfType(). These methods will return only one game object. Additionally, it is also possible to find all the game objects that have the same tag or are in the same type, using GameObject.FindGameObjectsWithTag( ) and Object.FindObjectsOfType( ). These methods will return arrays of game objects.
Contents
- Referencing game objects in Unity3D
- How to find game objects by their names
- Finding a game object that has a specific tag
- Getting the array of all game objects that have the same tag
- Finding a game object that matches a specific type
- Getting the array of all game objects that matches a specific type
- How to find a child game object
- Further remarks
Referencing game objects in Unity3D
What is referencing?
Most of the time, we want to access other game objects and their components. Hence, we can gather information about them when it is required, send information to them, control their behaviors, or execute a method that is in a script that is attached to them. In these cases, we need an address(or let’s say a phone number) of the game object and thus, we can dial whenever we want. This is called referencing.
While developing games in Unity3D, we always reference other game objects or components. Therefore, this is a fundamental topic you should understand before you go further.
How to create references to game objects or components
First of all, we have to declare variables that will store the address of the game objects or components. And hence, whenever we would like to access the properties of these game objects or components, we use these variables.
GameObject myGameObject; GameObject herGameObject; GameObject hisGameObject;
In the code above, we declared two variables in type GameObject but we have not assigned any object them yet. You may consider this as if we are reserving empty rows in an address book that we will fill them out later.
To reference these variables, we have a couple of options. We can search the game object that we want to assign using built-in methods that are included in Unity. We will see this option in later sections of this article. Another option is to assign relevant game objects directly in the Unity editor. But to do this we have to declare the objects either public or private with [SerializeField] attribute.
GameObject myGameObject; public GameObject herGameObject; [SerializeField]private GameObject hisGameObject;
In the code above, the first variable is declared as private(you can put the private keyword in front of it as well if you want), the second variable is declared as public and the third variable is declared as private with [SerializeField] attribute. [SerializeField] attribute makes this variable visible in the editor but still inaccessible from other scripts.
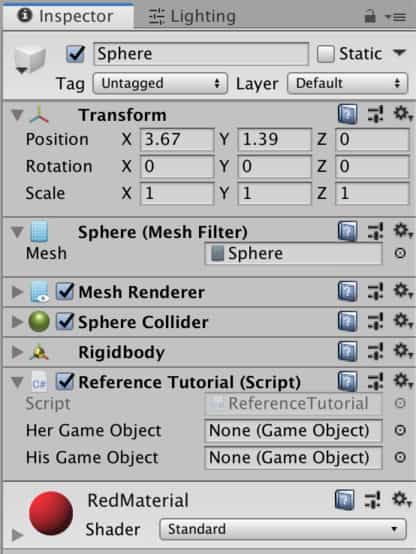
Now, you can drag and drop the game objects, that you would like to assign, to the slots that are visible in the inspector.
If you would like to create references to the components that are associated with the game objects, you need to declare variables that are in the type of that component.
Rigidbody rigidbodyComponent; BoxCollider boxColliderComponent;
How to find game objects by their names
As I mentioned above, we can create references to game objects and components by searching and finding them using built-in methods in Unity. This is useful especially when you want to stay the variable private or when you want to access an object that is created during runtime.
In order to search for a game object that has a specific name in the scene, we have to use the method GameObject.Find( ). It takes a string parameter. And this parameter is the name of the game object that we want to find.
myGameObject = GameObject.Find("Sphere");
In the code above, we created a reference for the game object that has the name “Sphere”.
If you would like to access a component that is attached to this game object, you should create a reference for that component. For instance, the following creates a reference for a rigidbody component that is attached to this game object, and hence you can access the properties of this component.
rigidbodyComponent = GameObject.Find("Sphere").GetComponent<Rigidbody>();
Finding a game object that has a specific tag
In addition to finding an object by its name, we can also find it by its tag which we are able to determine objects in the scene.
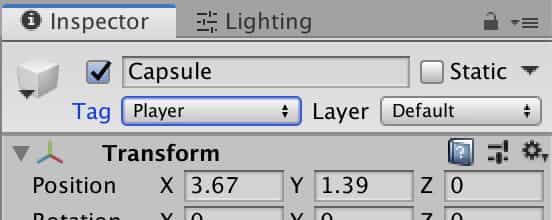
To find a game object that has a specific tag, we use the method GameObject.FindWithTag( ). This method takes a string parameter and searches for it (There is one more method that does the same job. You can also use GameObject.FindGameObjectWithTag( ) for the same purpose).
myGameObject = GameObject.FindWithTag("Player");
A tag can be used either for only one object or multiple objects. If there is more than one object that has the same tag, this method returns only one of them.
Getting the array of all game objects that have the same tag
If there are multiple objects that have the same tag and we want to get them all, we need to use the method GameObject.FindGameObjectsWithTag( ). Likewise, this method also takes a string parameter and returns an array of all game objects. Therefore, we have to declare an array that will store the game objects.
GameObject[] cubes;
The following returns and assigns all objects that have the tag “Cube”.
cubes = GameObject.FindGameObjectsWithTag("Cube");
Finding a game object that matches a specific type
We can also search and find a game object that matches a specific type. In other words, for instance, we can get a game object or component that has a specific component. To do this, we use the method Object.FindObjectOfType( ). This method also returns only one of the objects.
The following one finds a light component and creates a reference to it.
Light mainLight = (Light)FindObjectOfType(typeof(Light));
Getting the array of all game objects that match a specific type
If there are multiple game objects that match a specific type, we can find all of them and create references in an array. For this purpose, we use the method Object.FindObjectsOfType( ). This is an example of how we use it:
var sphereCollider = FindObjectsOfType(typeof(SphereCollider));
How to find a child game object
In order to find a child object and create a reference to that child object we can use the method Transform.Find( ). This method takes a string parameter and returns the child game object that the name matches this parameter.
Assume that there is a game object in the scene that has a tag “Player”. And also assume that this player object has a child game object which has the name “Gun”. If we want to create a reference for the “Gun” object, we may do this as the following:
GameObject gun=GameObject.FindWithTag("Player").transform.Find("Gun");
This is useful especially if there are multiple objects that has the same name under different objects.
Further remarks
In this article, we see different ways of how we reference game objects in Unity3D. Here, I need to warn you that searching and finding methods are extremely slow and you should avoid using them in Update, FixedUpdate, or LateUpdate methods, if possible. We generally use them in Start or Awake methods. You may also consider creating references in Singleton’s to improve performances. We have various tutorials about the Unity3D Engine that you can see in this link.